Querying Data and Data Sources with code and LINQ
To get started, we recommend you read the LINQ Guide and play around with the Razor Tutorial App
These common (extension) methods can be used on lists from 2sxc data streams (they all inherit from types implementing IEnumerable):
- .Where() - filter a list (IEnumerable) based on a specific criteria
- .Any() - returns true if any of the elements matches a criteria
- .OrderBy() / .OrderByDescending() - order a list (IEnumerable) by a specific field
- .First() / .Last() - get first or last element in the list
- .Select() - transform list into a new list, selecting specific field(s)
- .Take() / .Skip() - paging functions
- .Count() - count number of elements
- .IndexOf() - find index of a specific element in the list
For a full list of all System.Linq
methods, check out the methods of IEnumerable
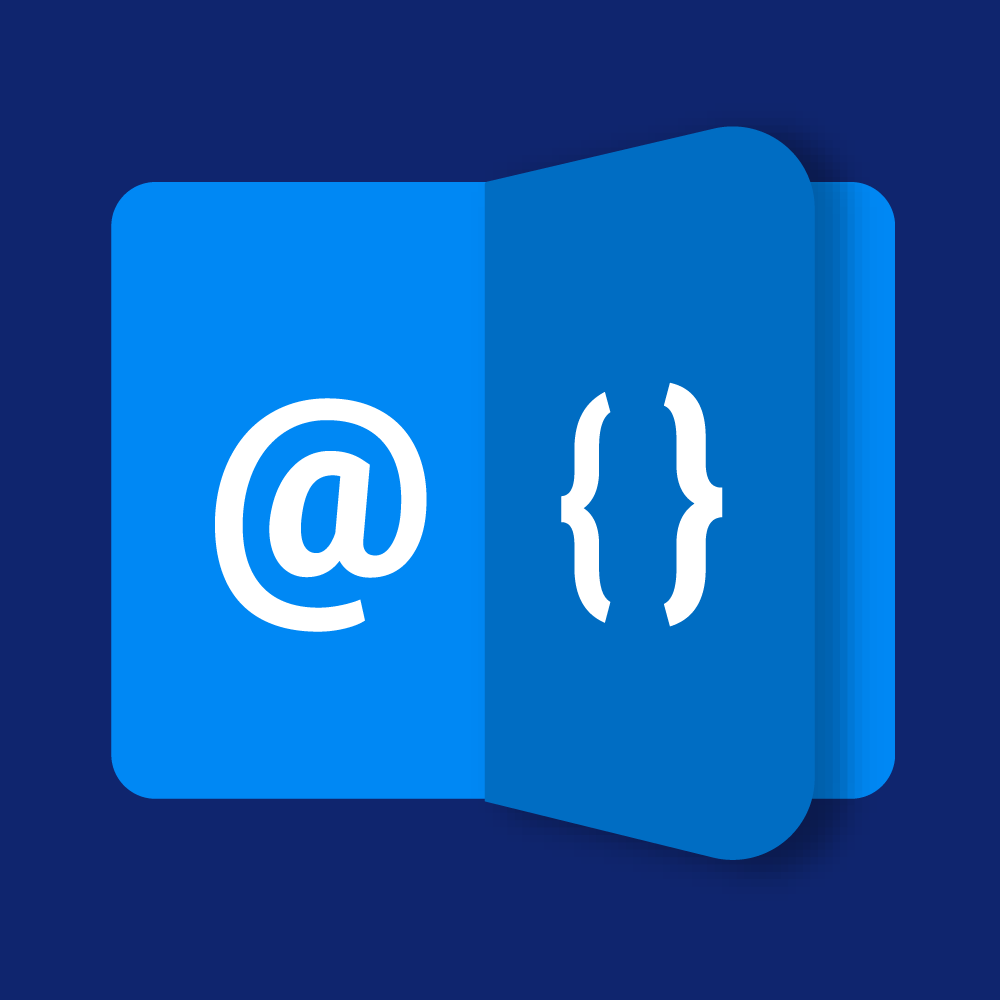
We have an rich series of Razor tutorials. You should really check them out 👍.
Using Statements
As explained in the guide we recommend the following using statements in Razor:
@using System.Linq;
@using Dynlist = System.Collections.Generic.IEnumerable<dynamic>;
Where
Filter a list (IEnumerable) based on a criteria.
Example: Basic filter of a list by string
var items = AsList(Data["Default"]);
items = items.Where(p => p.Name == "Chuck Norris");
// items now contains only items which have "Chuck Norris" as name property
Any
Returns true if any of the elements matches a criteria.
var items = AsList(Data["Default"]);
var containsChuckNorris = items.Any(p => p.Name == "Chuck Norris");
// if containsChuckNorris is true, at least one element has name "Chuck Norris"
Here's another Any - to see if a relationship contains something. It's a bit more complex, because Razor needs to know what it's working with:
// filter - keep only those that have this Category
// note that the compare must run on the EntityId because of object wrapping/unwrapping
postsToShow = postsToShow
.Where(p => (p.Categories as List<dynamic>)
.Any(c => c.EntityId == ListContent.Category[0].EntityId))
OrderBy / OrderByDescending
Order a list (IEnumerable) by a specific field.
var items = AsList(Data["Default"]);
items = items.OrderBy(p => p.Name);
// items are now ordered by property "Name"
First / Last
Get first or last element of the list.
var items = AsList(Data["Default"]);
var first = items.First(); // contains the first element
var last = items.Last(); // contains the last element
Select
Transform list into a new list, selecting only specified field(s).
var items = AsList(Data["Default"]);
var names = items.Select(p => p.Name); // names is a list of all names
Take / Skip
Paging functions: Take only n elements, skip m elements
var items = AsList(Data["Default"]);
items = items.Skip(10).Take(10); // Skips the first 10 elements and select only 10
Count
Count number of elements in a list.
var items = AsList(Data["Default"]);
var count = items.Count(); // contains the number of elements in the list
IndexOf
Find index of a specific element in the list.
@{
var items = AsList(Data["Default"]);
}
@foreach(var item in items) {
<h1>Item number @items.IndexOf(item)</h1>
}