C# Base Classes
Every C# helper file / custom service inherits from a base class. depending on the base class, the APIs and features in the template will change.
Example for /AppCode/Services/FunnyService.cs
- v17.03+:
namespace AppCode.Services
{
public class FunnyService : Custom.Hybrid.CodeTyped
{
public string PageTitle => MyPage.Title;
}
}
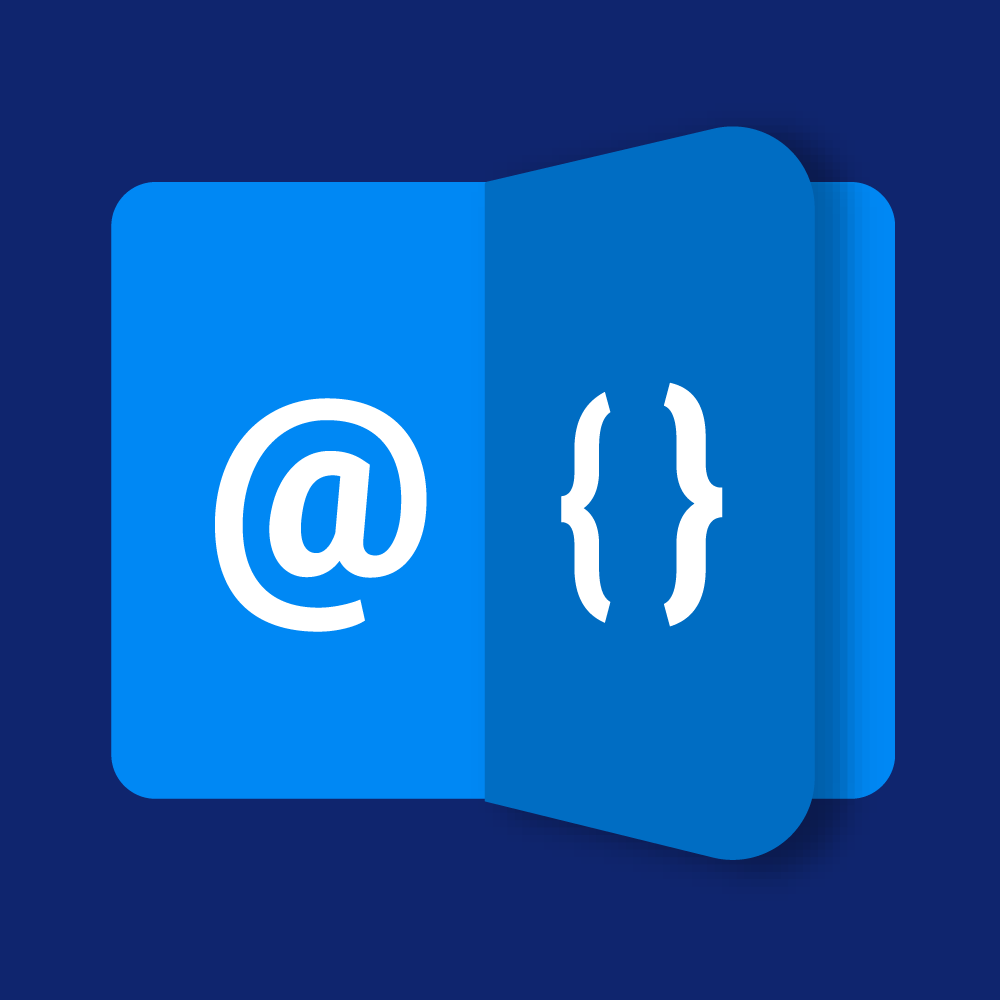
We have an rich series of Razor tutorials. You should really check them out 👍.
Typed / Strong-Typed for v16+
These are the recommended Typed C# base classes to inherit today:
Custom.Hybrid.CodeTyped
- new in v16
Tip
Using these base classes ensures that you have the latest, Typed APIs.
For older base classes and the differences, see below.
Older Base Classes (Dynamic)
These are the older - Dynamic base classes - not recommended for new development, but still supported for backwards compatibility.
Custom.Hybrid.Code14
- new in v14Custom.Hybrid.Code12
- new in v12Custom.Dnn.Code12
- v12; same as Hybrid, but withDnn
property
C# Classes Which Don't Inherit
If you don't specify a base class, the class will behave as a standard, new class. Example:
namespace AppCode.Services
{
public class FunnyService
{
// This can't work, because there is no MyPage object
public string PageTitle => MyPage.Title;
}
}
Compare Dynamic vs. Typed C# Code
The new Typed base classes are much more robust and easier to debug than the classic Dynamic code. They provide great IntelliSense (when configured in VS Code).
When used in combination with Data Models and Services in the AppCode folder, they also allow you to go Strong Typed. This is the recommended way to write code in 2sxc 16+.
Custom.Hybrid.CodeTyped
[!include["base-typed"](_base-typed_.md)]Base Classes in the AppCode Namespace
These are base classes for which the code lies in the AppCode/*
folder of your App.
Some will be auto-generated, others will be made by you.
📖 Read about the Typed API here TODO:
Custom.Hybrid.Code14
Note
Code14
ist the last release for Dynamic API.
It and all previous versions use Dynamic API.
See TODO:
Code14 almost identical with the older Code12 (see below) with these differences:
the
Kit
property is new, providing access to the ServiceKit called ServiceKit14. It provides access to all the services you need, likeData
,Security
,Koi
,Convert
and more.the
Convert
property is removed, as it caused confusion withSystem.Convert
Note: hybrid base classes don't have a Dnn
property. ...more
Custom.Hybrid.Code12 & Custom.Dnn.Code12
This was introduced in 2sxc 12. It contains the features which will work cross-platform on both Dnn and Oqtane. You should use this base class to create solutions / Apps which work on Dnn and Oqtane.
Custom.Dnn.Code12
is identical to Custom.Hybrid.Code12
but with the addition of the Dnn
property.
See also Dnn Object.
Limitations of Code12
Since this base class is meant to work on both Dnn and Oqtane, it only supports features which both of these platforms support.
The property
Dnn
doesn't exist on this base class, as it would lead to code which can't run cross-platform. ...moreThe
CreateInstance(...)
works only on C# files.cs
but not with CSHTML files.cshtml
as this probably won't work in .net 5Koi works differently than before. Previously you just used a global object
Connect.Koi.Koi
to use Koi, but because .net 5 should really use dependency injection, you should now get Koi usingGetService<Connect.Koi.ICss>()
. The old mechanism will still work in Dnn but would not work in Oqtane.