Custom DataSources
If you want to create your own DataSource and use it in C# or the VisualQuery designer, this is for you.
Get Started
🎓 Start with our Custom DataSource Guide
🗺️ Continue with the Big Picture
🚀 Then start to Create DataSources
For everything else, continue with the buttons in the menu to the left...
Use in VisualQuery Designer
This is what the DataSource would appear like in VisualQuery
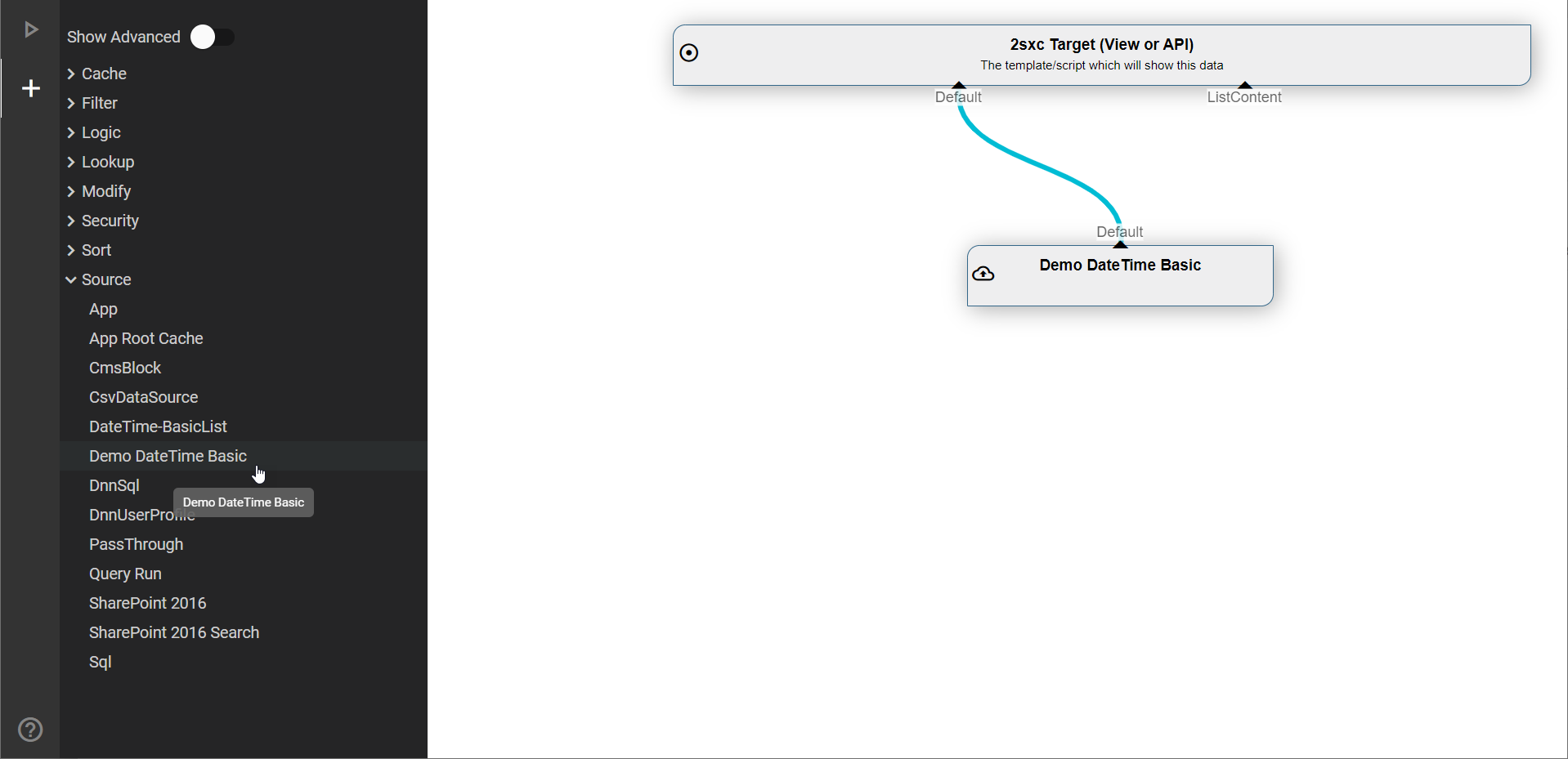
...and this is what the test-run would look like
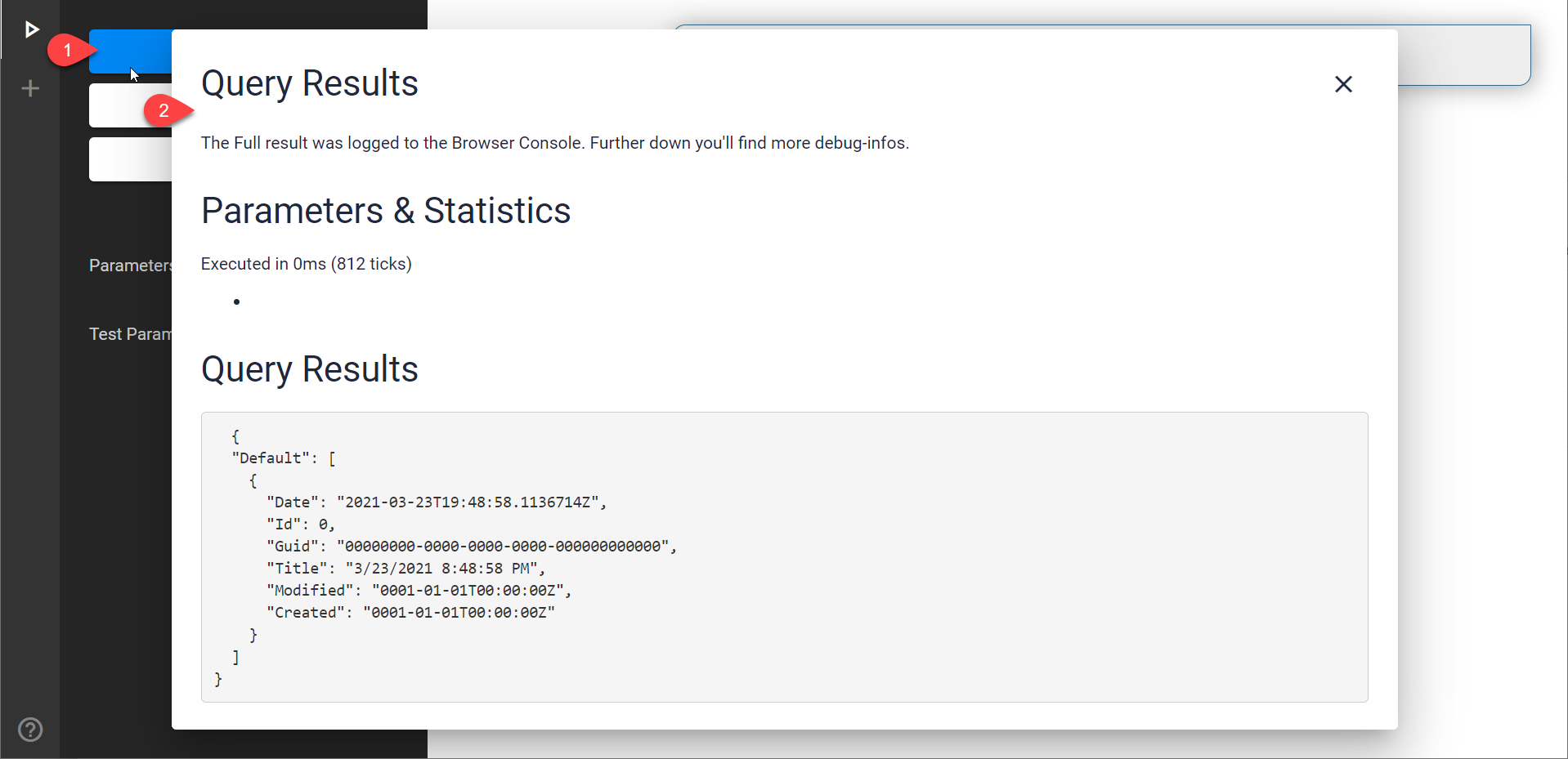
Read More
History
- Introduced in 2sxc ca. 4 but with a difficult API
- API strongly enhanced and simplifield in 2sxc 09.13
- Another API rework ca. 2sxc 10.25 (but we're not exactly sure)
- Major breaking API changes and improvements in 2sxc 15
- Dynamic DataSources introduced in 2sxc 16
Shortlink: https://go.2sxc.org/DsCustom