Dynamic Entity Properties
Whenever you create a content-type - like Person - and want to work with the data in your C# Razor templates, you'll be working with a Dynamic Entity. You can get any value using one of the following:
- the dot-notation like
Content.FirstName
- the
Get()
method likeContent.Get("FirstName")
- the
Get()
method with a fallback value likeContent.Get("FirstName", fallback: "John")
- the typed
Get<T>()
method likeContent.Get<string>("FirstName")
👉 also read about AsDynamic(...)
Code example using a Dynamic Entity
We'll assume we have a Content Type called Book with the following properties:
- Title (text, 1-line)
- Teaser (text, multi-line)
- Description (text, html)
- ReleaseDate (date)
- Author (entity - another content item)
Here's a code example in a C# Razor template:
<!--
The default variable for the current item is Content,
we'll just use another name for this sample
note that .Title is automatically provided,
because the content-type has the property title.
-->
<h1>@Content.Title</h1>
<div>@Content.Description</div>
<div>Author: @Content.Author.FullName</div>
So basically all properties of this book can be shown using [Object].[PropertyName]
- for example Content.ReleaseDate
.
What Dynamic Entity really does - and how
Technically the dynamic entity object is like a read-helper for the more complex IEntity. So actually the dynamic entity object will keep a reference to the underlying read-only IEntity
item on a property Entity
, and whenever your code accesses a property, the dynamic entity will query it from the underlying Entity
.
The main things that the dynamic entity does for you, are
- Give you a nice, short syntax to access a property - so
Content.FirstName
instead ofObject.Attributes["FirstName"]["en"]
which would be necessary using the more advancedIEntity
object - Ensure that the language used in retrieving a value is the current user language
- Give conveniant access to related information like the
Presentation
object - Automatically handle some data-not-found errors
- Automatically do conversions, like convert related entities (like
.Children
) into dynamic objects to make your code more consistant
How the Property Lookup Works
Internally there are a few things that can returned if you do something like Content.SomeProperty
- If the
SomeProperty
is one of the internal properties likeEntityId
etc. (see below) this will be returned - Next is a simple property of the underlying Entity,
- like
FirstName
which would be a string - or a relationship property like
Tags
which will return a special DynamicEntity that behaves as a list (see below)
- like
- Last but not least - if nothing matches, it's
null
Properties of a Dynamic Entity
Read the API docs in the IDynamicEntity.
Additional properties that work (they are dynamic, so don't appear in the code)
- EntityId int
- EntityGuid Guid
- EntityType string - the type name like Person
- IsPublished bool - true/false if this item is currently published
- AnyProperty dynamic, but actually bool | string | decimal | datetime | List
any normal property of the content-item can be accessd directly. It's correctly .net typed (string, etc.)
Tip
In 2sxc 10.27 any property that returns a List<DynamicEntity>
now returns a IDynamicEntity containing a list.
This means that if you expect the list to just return one item, you can directly access its properties like this:
Content.Author.FirstName
.
To otherwise enumerate the items, we recommend AsList(object) so AsList(Content.Tags)
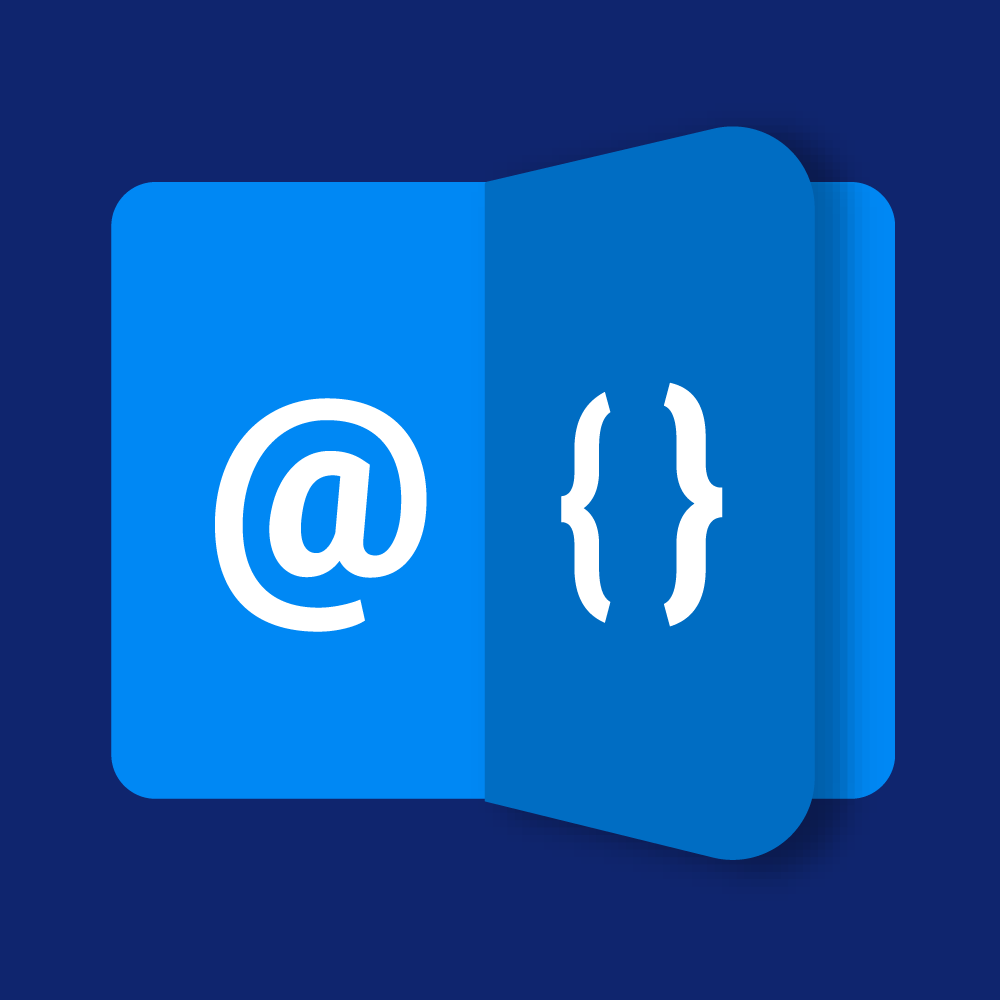
We have an rich series of Razor tutorials. You should really check them out 👍.
Appendix
The following properties/methods exist, but shouldn't be used. They are documented here so that you know that they are not meant for public use:
- Created - the created date
- Modified - the modified date
- Owner - the current owner of the item, usually the author
- Metadadata - currently use
AsEntity(theObject).Metadata
- Permissions - permissions of the current item (if any are defined)
History
- Introduced in 2sxc 01.00
- Changed to use interface IDynamicEntity in 6.x
- Draft/Published introduced in 2sxc 7.x
- Presentation introduced in 2sxc 7.x
- Modified introduced in 2sxc 8.x
- Implemented .net equality comparer in 2sxc 9.42
- Parents added in 2sxc 9.42
- Get added in 2sxc 9.42 and added to interface IDynamicEntity in 10.07
- Parents introduced in 2sxc 9.42, and added to interface IDynamicEntity in 10.07
- IsDemoItem property added in 2sxc 10.06
- Changed dynamic access to a property to return a DynamicEntity which is enumerable in 10.27