Dynamic Entity Lists
It's very common to work with a list of items, like a list of blog posts, tags etc.
2sxc has a lot of magic under the hood to just make it work. Here's an example:
<ul>
@foreach(var tag in blogPost.Tags) {
<li>@tag.Name</li>
}
</ul>
To get really good at coding lists, there are a few things you want to learn:
- Use list from different sources
- Entities which are a property of something (like
blogPost.Tags
) - Entities which belong to the Module
- Entities which come from a Query
- Entities of a specific Content-Type from App.Data
- Entities which are a property of something (like
- Difference between Entity-lists and DynamicEntity-Lists
- Looping
- Using LINQ to sort, filter and more
Basics First - What Are Lists of Dynamic Entities
In the lingo of C# they are IEnumerable<IDynamicEntity>
objects.
But basically lists are objects that can be stepped through (iterated).
You will usually use them to show the list of items (like a list of News items). And if the list has too much data or is in a weird sorting order, you'll usually want to filter and sort before doing this.
How to Get a List of Dynamic Entities
In many cases the list is aleady there for you to use.
For example, if your BlogPost
object has a property Tags
which is an Entity-Picker in the Edit-UI, then this will automatically work:
<ul>
@foreach(var tag in blogPost.Tags) {
<li>@tag.Name</li>
}
</ul>
In other scenarios you may get objects which are still IEntity objects. For example, App.Data["BlogPost"]
will get you a list of IEntity
objects.
But these don't allow you to just access a property, so you'll have to use AsList(...)
.
@* this won't work *@
- Find some information
Whenever you create a content-type - like Person - and want to work with the data in your C# Razor templates, you'll be working with a Dynamic Entity.
👉 also read about AsDynamic(...)
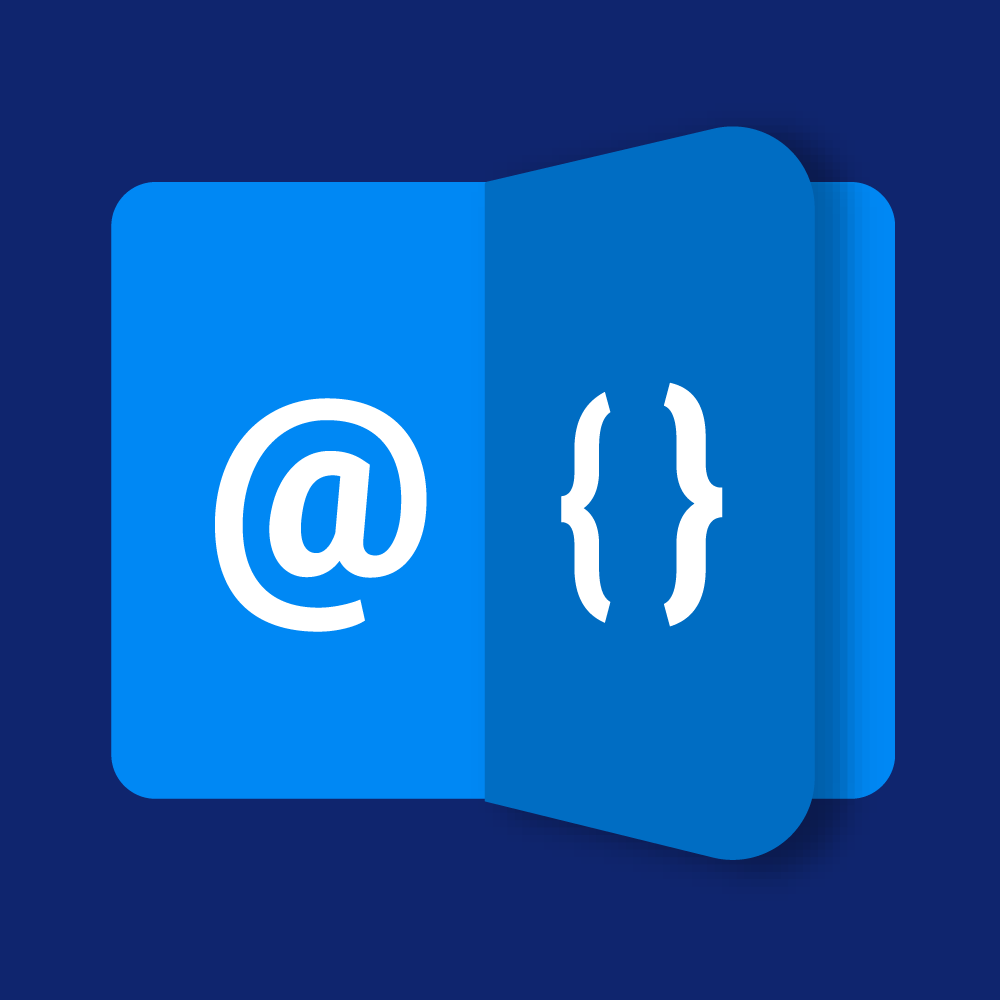
We have an rich series of Razor tutorials. You should really check them out 👍.
History
- Introduced in 2sxc 01.00
- Changed to use interface IDynamicEntity in 6.x